What is SQL Injection? Hack a Website Database Attack Online
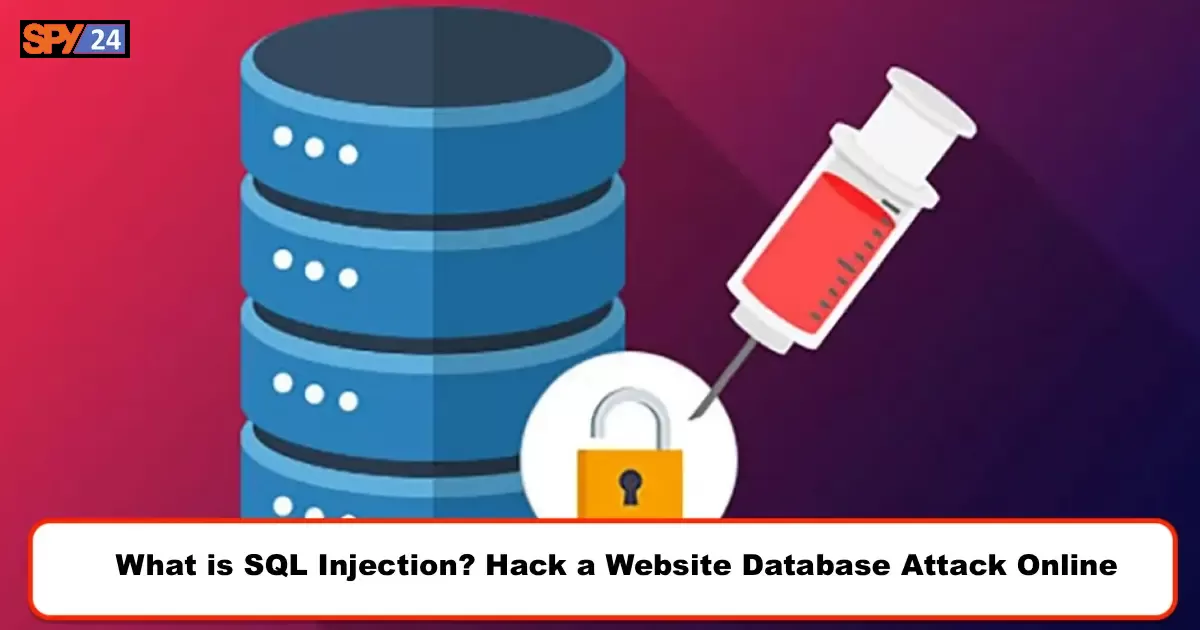
A SQL injection attack, or SQLI, is a way of getting access to information that wasn’t supposed to show up on a site by manipulating the backend database with malicious SQL code. Information like this can be sensitive about a company, customer lists, or customer info.
An SQL injection can ruin your business. When someone is hacking into an organization, their users can be viewed by an unauthorized person, entire tables can get deleted, and a malicious person can access even the whole database. All that can hurt the business of the organization.
You should factor in the likelihood that you might lose your customers’ trust when calculating the cost of an SQLi when their personal information may be compromised, such as passwords, phone numbers, or credit card details, when calculating the cost.
Sites are the most common place to attack SQL databases because of the variety of vulnerabilities and vectors.
SQL injection examples
A SQL injection vulnerability or attack can occur in many situations and can have applications in a bunch of different fields. SQL injection examples include:
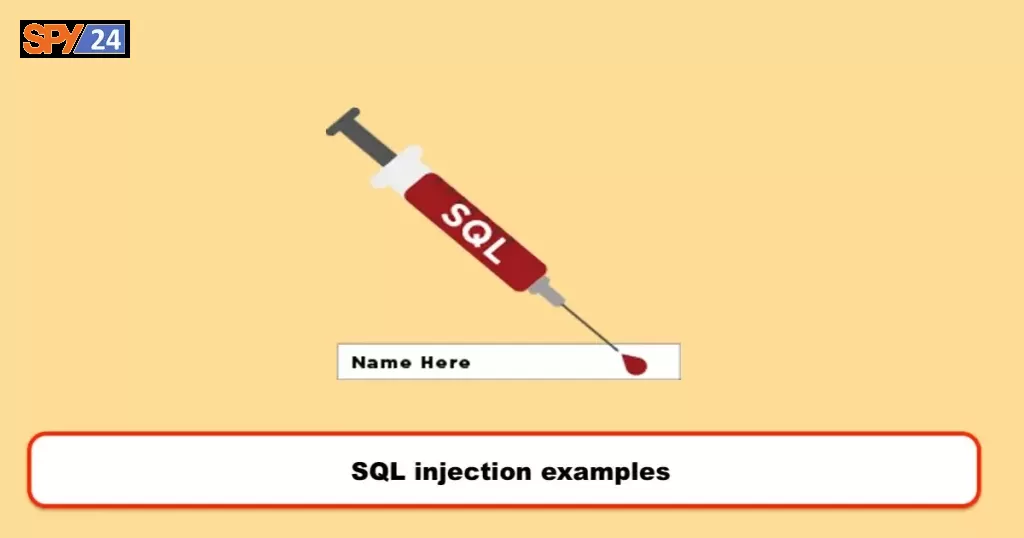
- Retrieving hidden data, where you can modify an SQL query to return additional results.
- Subverting application logic, where you can change a query to interfere with the application’s logic.
- UNION attacks, where you can retrieve data from different database tables.
- Examining the database, where you can extract information about the version and structure of the database.
- Blind SQL injection, where the results of a query you control are not returned in the application’s responses.
Retrieving hidden data
When a user clicks on the Gifts category in a shopping application, their browser requests the following URL:
https://insecure-website.com/products?category=Gifts
This will be followed by the execution of a SQL query at this point to retrieve all the details related to the products that are required:
SELECT * FROM products WHERE category = ‘Gifts’ AND released = 1
This SQL query asks the database to return:
- all details (*)
- from the products table
- where the category is Gifts
- and released is 1.
In this case, released equal to 1 will be used as a restriction to hide the products that have not been released yet. In the case of unreleased products, released equal to 0 is used.
As there is no SQL injection defense built into the application, an attacker can take the following approach in order to exploit it:
https://insecure-website.com/products?category=Gifts’–
The result of the SQL query will be:
SELECT * FROM products WHERE category = ‘Gifts’–‘ AND released = 1
As a result, the query that follows will be interpreted in SQL as a comment, since the double-dash sequence indicates that the query should be interpreted as a comment. Due to this, the query is effectively removed since it does not contain the keyword AND released equals one, which enables all products to be viewed, even those that have not yet been released.
Furthermore, they can display any product in any category, even categories they don’t know about because the application displays everything:
https://insecure-website.com/products?category=Gifts’+OR+1=1–
This results in the SQL query:
SELECT * FROM products WHERE category = ‘Gifts’ OR 1=1–‘ AND released = 1
The modified query will return all items where either the category is Gifts, or 1 is equal to 1. Since 1=1 is always true, the query will return all items.
Subverting application logic
If a user is allowed to log in with a username and password, the application will use the following SQL query to verify his credentials: Let’s say he can log in with these credentials. If the user enters baldeagle as their username and blueberry as their password, the application checks their credentials as follows:
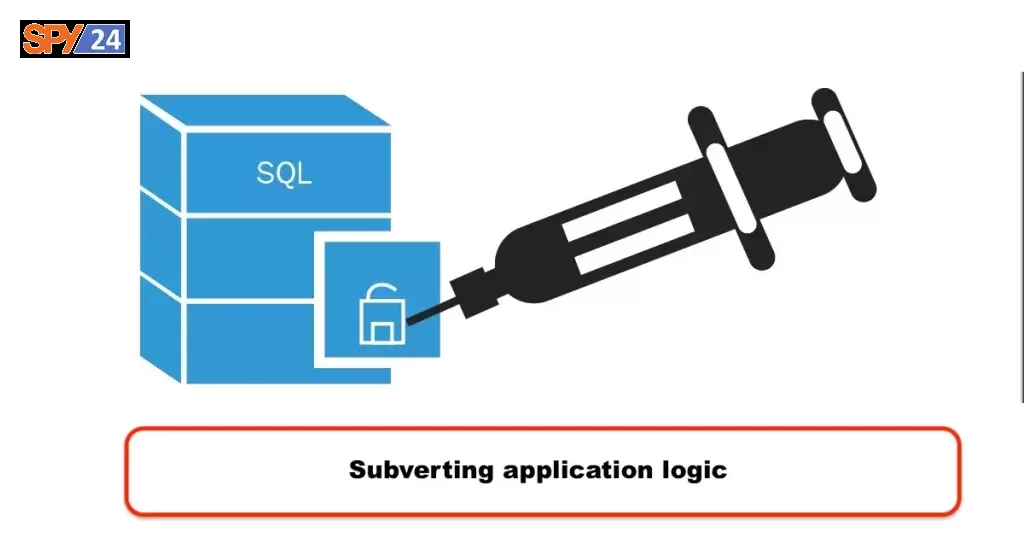
SELECT * FROM users WHERE username = ‘baldeagle’ AND password = ‘blueberry’
In case of a successful login, the user’s details would be returned. Otherwise, it would be expected that the login failed.
Using the SQL comment sequence above, if an attacker forgets the password check you put in before submitting administrator, they can log in with any blank password as any user if they forget the password check you added to the WHERE clause. To let users without passwords log in, submit administrator without a password. The following query, for example, gets results when the administrator is logged in with no password.
SELECT * FROM users WHERE username = ‘administrator’–‘ AND password = ”
In this case, the query returns a user whose username is administrator, and the attacker is able to successfully log in as that user via the query.
Retrieving data from other database tables
You can use a SQL injection vulnerability to get data from other tables in the database if query results are returned within the application’s responses. By using the UNION keyword, a second SELECT query can be executed to append the original query’s results, making this easy.
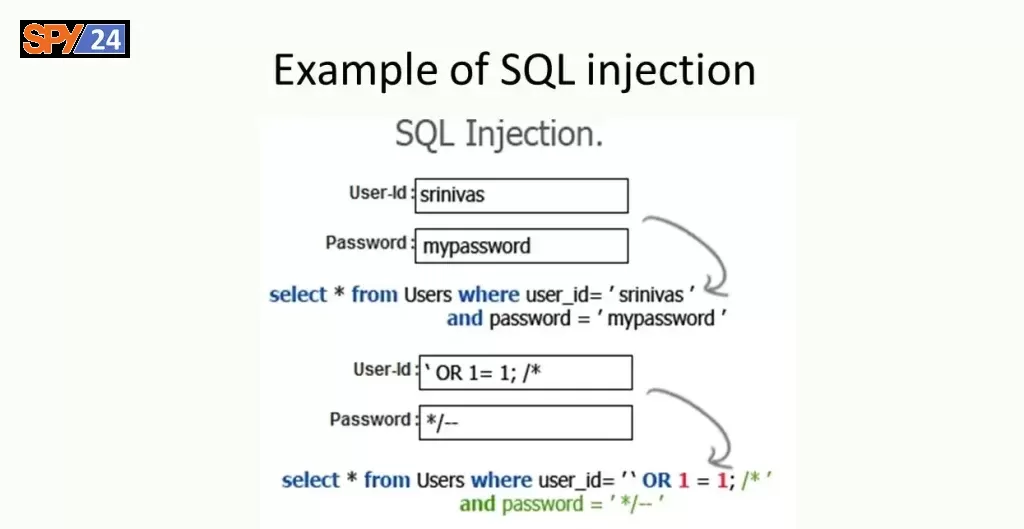
When an application runs this query, with the user input “Gifts,” it shows these results:
SELECT name, description FROM products WHERE category = ‘Gifts’
Input can then be submitted by an attacker as follows:
‘ UNION SELECT username, password FROM users–
You can get all the usernames, passwords, and product names, and descriptions along with the usernames and passwords for this user.
Examining the database
Often vulnerability hunters are interested in the database itself when they find an SQL injection vulnerability, so they can get some information about it.
It’s easy to find out what kind of database you have, depending on the database type you use, so use the method that yields the best results. Here’s an Oracle query for searching version details:
SELECT * FROM v$version
A query can tell you what columns each table in a database has, as well as tell you which tables are in the database. For example, on most databases, this query will tell you which tables are in the database:
SELECT * FROM information_schema.tables
Blind SQL injection vulnerabilities
A blind injection vulnerability is one that can only be exploited when the application doesn’t give back SQL query results, or any details about database errors, which means it’s blind. But blind vulnerabilities can still be exploited, but they’re usually much more complex and challenging to use.
Blind SQL injection vulnerabilities can be exploited with lots of different techniques, depending on the vulnerability and the database:
- By changing the query logic, the application will react to a certain condition in a different way, triggering a change in how the query works. The result is usually a condition injected in a Boolean logic formula or a conditional error, like dividing by zero.
- When the condition is satisfied, the application will process the query, and the time it takes to respond can be used to test the condition’s truthiness.
- It is possible to generate out-of-band network interactions using OAST methods. The out-of-band network interaction can be extremely powerful and provide solutions for situations that are not currently available. Using the out-of-band channel makes exfiltrating data easier. For example, you can use a DNS lookup for the domain you control to place the data in.
How to detect SQL injection vulnerabilities
You can identify web SQL injection vulnerabilities with Burp Suite’s web vulnerability scanner quickly and easily.
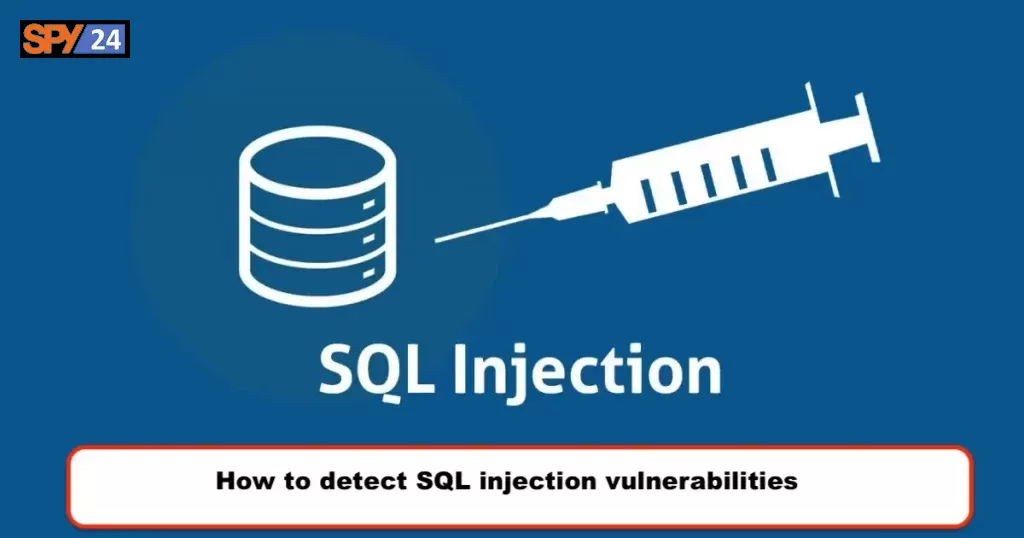
It is possible to detect SQL injection manually by performing systematic and methodical testing against all the entry points in the application. These tests usually include:
- You can check for errors or anomalies by entering ‘.
- It’s easy to tell if values are different if you submit some SQL syntax that evaluates the default entry point value and the corresponding value of another entry point.
- You should combine Boolean conditions like OR 1 = 1 and OR 1 = 2, as well as inferred differences between responses.
- Using a payload that triggers a time delay within an SQL query, we’re observing the difference in query response times.
- The submission of payloads that trigger out-of-band network interactions and monitoring of the outcome of those interactions.
How to prevent SQL injection
A parameterized query (or prepared statement) protects a computer from SQL injection attacks since the query does not contain concatenated strings within it like other queries normally do.
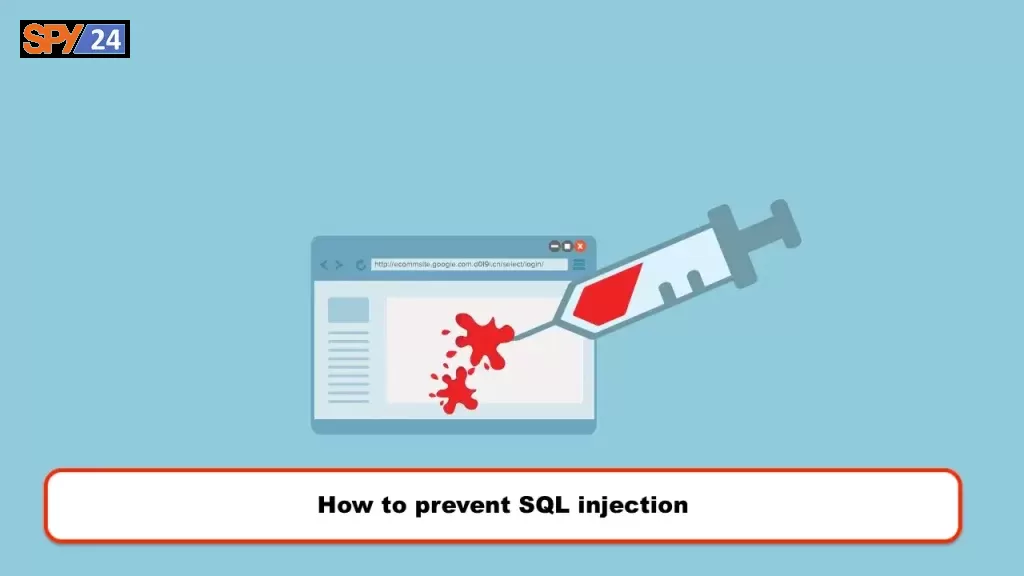
It is believed that the code below increases the risk of SQL injection since user input is directly concatenated with the query in the following way:
String query = “SELECT * FROM products WHERE category = ‘”+ input + “‘”;
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery(query);
If you rewrite the code below, user input won’t interfere with query flow and it won’t need to be rewritten substantially:
PreparedStatement statement = connection.prepareStatement(“SELECT * FROM products WHERE category = ?”);
statement.setString(1, input);
ResultSet resultSet = statement.executeQuery();
With parameterized queries, untrusted input can be used anywhere in the query that contains data, including WHERE clauses and INSERT or UPDATE statements with untrusted values.
Moreover, it has been suggested they cannot be used in other places, such as in an “Order by” clause or a table name, since they are not trusted for handling untrusted input for other things.
The applications can be configured to whitelist permissible input values so that no untrusted data can be entered into those areas of the query, or they can alter their logic to accomplish what they need to do.
A parameterized query in an application should always contain a constant string, and should never contain any variable data, no matter where it comes from. This will prevent SQL injection.
The string concatenation should remain the same if the data is trustworthy, so you don’t need to make case-by-case decisions as to whether or not the data needs to be trusted according to your query.
Moreover, errors relating to the origin of tainted data, as well as changes made to another code that contradict assumptions about tainted data, can affect the interpretation of the data.
SPY24 Install application free The Most Powerful Hidden Spying App to Monitor Android, IOS Cell Phone & Tablet Device Remotely. Best Android Parental Control App for Kids & Teens Online Safety.
Now take the liberty to monitor Android, and IOS devices with exclusive features better than ever before Monitor all Voice & Text Messages Communication records, Listen to & Watch Surroundings in Real-time Unleash Digital Parenting with Android, IOS Spy App Spy (Surround Listing & Front/Back Camera Bugging) IM’s VoIP call recording on Android OS 13 & above With 250+ Surveillance Tools at your fingertips using Android Tracking & Parental Monitoring Software.